

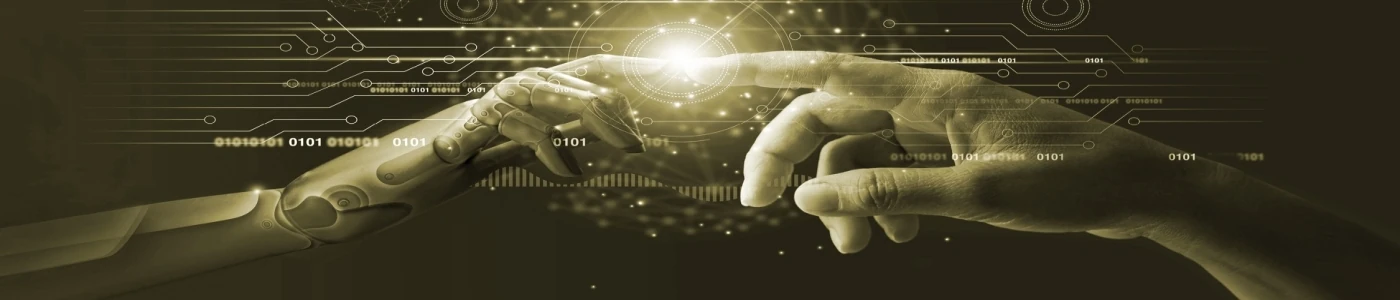

S
SciLor's HD2 / Leo Multitouch .NET CF DLL
Erstellt
![]() |
letzte Antwort | Antworten
4
|
Aufrufe
7.1T |
15
„Gefällt mir“ |
Abos
1 |
Do., 06. Mai, 2010 um 20:05
#1
SciLor's .NET CF MultiTouch DLL v0.1 adds MultiTouch-Support to your existing .NET CF software very easily (Currently only for the HD2): If I release a new DLL, it WILL be fully compatible to the previous one! First of all you will have to add your app to the PinchToZoom Whitelist in the registry: Code: [HKEY_LOCAL_MACHINE\Software\HTC\TouchGL\Pinch\WhiteList\YourApp] "ProcName"="YouAppProcessName.exe" After adding, soft reset! Then add the "SciLors-MultiTouch.dll" as reference in your Visual Studio project. Beware, it is not allowed to change the assembly name! It must stay "SciLors-MultiTouch.dll" or it will not work! Afterwards declare the MultiTouch Class: VB.NET: Code: Dim myMultiTouch As New SciLorsMultiTouch.SciLorsMultiTouch C#: Code: SciLorsMultiTouch.SciLorsMultiTouch myMultiTouch = new SciLorsMultiTouch.SciLorsMultiTouch(); Then you add following to your Form_Load event: VB.NET: Code: Call myMultiTouch.CatchWndProc(Me) AddHandler myMultiTouch.MouseDown, AddressOf frmMain_MouseDown AddHandler myMultiTouch.MouseMove, AddressOf frmMain_MouseMove AddHandler myMultiTouch.MouseUp, AddressOf frmMain_MouseUp C#: Code: myMultiTouch.CatchWndProc(this); myMultiTouch.MouseDown += frmMain_MouseDown; myMultiTouch.MouseMove += frmMain_MouseMove; myMultiTouch.MouseUp += frmMain_MouseUp; Now, at every MultiTouch Event it gets delegated to your frmMain_Mouse* procedures (There will be always 2 Events fired due to there existing 2 Fingers on the screen ![]() Code: Single Finger: Windows.Forms.MouseButtons.None or MouseButton = Windows.Forms.MouseButtons.Left MultiTouch: Finger 1: Windows.Forms.MouseButtons.Middle Finger 2: Windows.Forms.MouseButtons.Right Code Examples: VB.NET: Code: Public Structure MouseState Dim Position As Point Dim MouseDown As Boolean End Structure Public Fingers(2) As MouseState Public Sub frmMain_MouseDown(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles frmMain.MouseDown Dim ButtonID As Byte = ButtonToID(e.Button) With Fingers(ButtonID) .Position.X = e.X .Position.Y = e.Y .MouseDown = True End With End Sub Public Sub frmMain_MouseMoveByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles frmMain.MouseMove Dim ButtonID As Byte = ButtonToID(e.Button) With Fingers(ButtonID) .Position.X = e.X .Position.Y = e.Y .MouseDown = True End With End Sub Public Sub frmMain_MouseUp(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles frmMain.MouseUp Dim ButtonID As Byte = ButtonToID(e.Button) With Fingers(ButtonID) .Position.X = e.X .Position.Y = e.Y .MouseDown = False End With End Sub Private Function ButtonToID(ByVal MouseButton As MouseButtons) If MouseButton = Windows.Forms.MouseButtons.None Or MouseButton = Windows.Forms.MouseButtons.Left Then Return 0 ElseIf MouseButton = Windows.Forms.MouseButtons.Middle Then Return 1 Else Return 2 End If End Function C#: Code: public struct MouseState { public Point Position; public bool MouseDown; } public MouseState[] Fingers = new MouseState[3]; public void pctDraw_MouseDown(object sender, MouseEventArgs e) { int ButtonID = Conversions.ToByte(this.ButtonToID(e.Button)); this.Fingers[ButtonID].Position.X = e.X; this.Fingers[ButtonID].Position.Y = e.Y; this.Fingers[ButtonID].MouseDown = true; } public void pctDraw_MouseMove(object sender, MouseEventArgs e) { int ButtonID = Conversions.ToByte(this.ButtonToID(e.Button)); this.Fingers[ButtonID].Position.X = e.X; this.Fingers[ButtonID].Position.Y = e.Y; this.Fingers[ButtonID].MouseDown = true; } public void pctDraw_MouseUp(object sender, MouseEventArgs e) { int ButtonID = Conversions.ToByte(this.ButtonToID(e.Button)); this.Fingers[ButtonID].Position.X = e.X; this.Fingers[ButtonID].Position.Y = e.Y; this.Fingers[ButtonID].MouseDown = false; } private object ButtonToID(MouseButtons MouseButton) { if ((MouseButton == MouseButtons.None) | (MouseButton == MouseButtons.Left)) { return 0; } if (MouseButton == MouseButtons.Middle) { return 1; } return 2; } Function Description/Documentation: -CatchWndProc(myForm, Optional PositionFixX, Optional PositionFixY): Redirects the Windows Messages from the "myForm" to the Multitouch Class. If you want to use it for a Control on top of the Form, the PositionFix can add/remove constant values to the returned MouseMessages. -RestoreWndProc(): Detaches the Multitouch Class from the Form to restore the normal Window Message flow. -UpdatePositionFix(PositionFixX, PositionFixY): If your control has changed it's position you can change its position in the class -UpdateFormTopLeft(PositionFixLeft, PositionFixTop): If the Top-Left Corner of the Form changes you may use this. -GetInformation(): Gives a Information String of the Multitouch DLL. It includes the version and some contact information. -Multiple Instances per Form are not supported and not needed (Multitouch events fire allways, everywhere on the form)! -The Mouse-Coordinates are allways relative to the forms top-left corner. ReadMe.txt (without code) Code: SciLor's .NET CF MultiTouch DLL v0.2 i. .7. .. :v c: .X i.:: : ..i.. #MMMMM QM AM 9M zM 6M AM 2M 2MX#MM@1. OM tMMMMMMMMMM; .X#MMMM ;MMMMMMMMMMMMv cEMMMMMMMMMU7@MMMMMMMMMMMMM@ .n@MMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMM MMMMMMMM@@#$BWWB#@@#$WWWQQQWWWWB#@MM. MM ;M. $M EM WMO$@@@@@@@@@@@@@@@@@@@@@@@@@@@@#OMM #M cM QM Another Cake by tM MM SciLor CMO .MMMM oMMMt 1MO 6MMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMM iMM .M1 BM For all Portal Lovers! vM ,Mt 1M @M............................... WM M6 MM .A8OQWWWWWWWWWWWWWWWWWWWWWWWWWWWOAz2 #M MM MM. @MMY vMME UMMMbi i8MMMt C@MMMMMbt;;i.......i;XQMMMMMMt ;ZMMMMMMMMMMMMMMM@A;. ReadMe You will need the .NET CF 2.0 This DLL adds MultiTouch-Support to your existing .NET CF software very easily (Currently only for the HD2): First of all you will have to add your app to the PinchToZoom Whitelist in the registry: [HKEY_LOCAL_MACHINE\Software\HTC\TouchGL\Pinch\WhiteList\YourApp] "ProcName"="YouAppProcessName.exe" After adding, soft reset! Then add the "SciLors-MultiTouch.dll" as reference in your Visual Studio project. Beware, it is not allowed to change the assembly name! It must stay "SciLors-MultiTouch.dll" or it will not work! Afterwards declare the MultiTouch Class: VB.NET: Dim myMultiTouch As New SciLorsMultiTouch.SciLorsMultiTouch C#: SciLorsMultiTouch.SciLorsMultiTouch myMultiTouch = new SciLorsMultiTouch.SciLorsMultiTouch(); Then you add following to your Form_Load event: VB.NET: Call myMultiTouch.CatchWndProc(Me) AddHandler myMultiTouch.MouseDown, AddressOf frmMain_MouseDown AddHandler myMultiTouch.MouseMove, AddressOf frmMain_MouseMove AddHandler myMultiTouch.MouseUp, AddressOf frmMain_MouseUp C#: myMultiTouch.CatchWndProc(this); myMultiTouch.MouseDown += frmMain_MouseDown; myMultiTouch.MouseMove += frmMain_MouseMove; myMultiTouch.MouseUp += frmMain_MouseUp; Now, at every MultiTouch Event it gets delegated to your frmMain_Mouse* procedures (There will be always 2 Events fired due to there existing 2 Fingers on the screen ;) ). You can easily detect wheather the mouse event comes from a normal mouse-press or a multitouch finger. Single Finger: Windows.Forms.MouseButtons.None or MouseButton = Windows.Forms.MouseButtons.Left MultiTouch: Finger 1: Windows.Forms.MouseButtons.Middle Finger 2: Windows.Forms.MouseButtons.Right Code Examples: VB.NET: Public Structure MouseState Dim Position As Point Dim MouseDown As Boolean End Structure Public Fingers(2) As MouseState Public Sub frmMain_MouseDown(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles frmMain.MouseDown Dim ButtonID As Byte = ButtonToID(e.Button) With Fingers(ButtonID) .Position.X = e.X .Position.Y = e.Y .MouseDown = True End With End Sub Public Sub frmMain_MouseMoveByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles frmMain.MouseMove Dim ButtonID As Byte = ButtonToID(e.Button) With Fingers(ButtonID) .Position.X = e.X .Position.Y = e.Y .MouseDown = True End With End Sub Public Sub frmMain_MouseUp(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles frmMain.MouseUp Dim ButtonID As Byte = ButtonToID(e.Button) With Fingers(ButtonID) .Position.X = e.X .Position.Y = e.Y .MouseDown = False End With End Sub Private Function ButtonToID(ByVal MouseButton As MouseButtons) If MouseButton = Windows.Forms.MouseButtons.None Or MouseButton = Windows.Forms.MouseButtons.Left Then Return 0 ElseIf MouseButton = Windows.Forms.MouseButtons.Middle Then Return 1 Else Return 2 End If End Function C#: public struct MouseState { public Point Position; public bool MouseDown; } public MouseState[] Fingers = new MouseState[3]; public void pctDraw_MouseDown(object sender, MouseEventArgs e) { int ButtonID = Conversions.ToByte(this.ButtonToID(e.Button)); this.Fingers[ButtonID].Position.X = e.X; this.Fingers[ButtonID].Position.Y = e.Y; this.Fingers[ButtonID].MouseDown = true; } public void pctDraw_MouseMove(object sender, MouseEventArgs e) { int ButtonID = Conversions.ToByte(this.ButtonToID(e.Button)); this.Fingers[ButtonID].Position.X = e.X; this.Fingers[ButtonID].Position.Y = e.Y; this.Fingers[ButtonID].MouseDown = true; } public void pctDraw_MouseUp(object sender, MouseEventArgs e) { int ButtonID = Conversions.ToByte(this.ButtonToID(e.Button)); this.Fingers[ButtonID].Position.X = e.X; this.Fingers[ButtonID].Position.Y = e.Y; this.Fingers[ButtonID].MouseDown = false; } private object ButtonToID(MouseButtons MouseButton) { if ((MouseButton == MouseButtons.None) | (MouseButton == MouseButtons.Left)) { return 0; } if (MouseButton == MouseButtons.Middle) { return 1; } return 2; } Function Description/Documentation: -CatchWndProc(myForm, Optional PositionFixX, Optional PositionFixY): Redirects the Windows Messages from the "myForm" to the Multitouch Class. If you want to use it for a Control on top of the Form, the PositionFix can add/remove constant values to the returned MouseMessages. -RestoreWndProc(): Detaches the Multitouch Class from the Form to restore the normal Window Message flow. -UpdatePositionFix(PositionFixX, PositionFixY): If your control has changed it's position you can change its position in the class -UpdateFormTopLeft(PositionFixLeft, PositionFixTop): If the Top-Left Corner of the Form changes you may use this. -GetInformation(): Gives a Information String of the Multitouch DLL. It includes the version and some contact information. ToDo: Additional: -Multiple Instances are not supported and not needed (Multitouch events fire allways, everywhere on the form)! -The Mouse-Coordinates are allways relative to the forms top-left corner. Known Bugs: If you like my hard work PLEASE DONATE! :) http://www.scilor.com/donate.html ChangeLog: v0.2 (2010-02-15) -Enhancement: Added "UpdatePositionFix"/"UpdateForm" and "RestoreWndProc" -Enhancement: Added some Documentation ----------------------- v0.1 (2009-12-07) -Initial Release ---------------LINKS------------------------- My Website: http://www.scilor.com/ SciLor's .NET CF MultiTouch DLL Website: http://www.scilor.com/hd2-leo-dotNetCfMultiTouchDLL.html Donation: http://www.scilor.com/donate.html - Zuletzt bearbeitet am 06.05.2010 20:06, insgesamt 1-mal bearbeitet |
|
Do., 06. Mai, 2010 um 20:06
|
|
Do., 06. Mai, 2010 um 20:29
#3
Das müsste das Programm aus dem Video sein... Kanns leider grad nicht testen weil mein Handy nicht bei mir ist ![]() |
|
Do., 06. Mai, 2010 um 22:44
#4
kefir hat Folgendes geschrieben: Das müsste das Programm aus dem Video sein... Kanns leider grad nicht en weil mein Handy nicht bei mir ist ![]() Ja ist es! Am Samsung Omnia konnte ich es installieren und es läuft auch.. Kommt aber mit Auflösung nicht zurecht.. ![]() Der Mensch ist ein naiver Tourist mit einem abgelaufenem Visum für den Planeten Erde .. |
|
Fr., 28. Mai, 2010 um 10:49
#5
aleX hat Folgendes geschrieben: Kommt aber mit Auflösung nicht zurecht.. ![]() kefir hat Folgendes geschrieben: SciLor's .NET CF MultiTouch DLL v0.1 adds MultiTouch-Support to your existing .NET CF software very easily (Currently only for the HD2): If I release a new DLL, it WILL be fully compatible to the previous one! ![]() |
|

Du hast bereits für diesen
Post abgestimmt...
;-)

Ähnliche Themen:
© by Ress Design Group, 2001 - 2024
